2. Overview
This document is written for summarizing my contributions in the project SaveIt.
SaveIt is geared at keeping track of the issues and solutions that the user finds online. It is designed for programmers who are used to Command Line Interface​ (CLI) applications and are good at typing while still having the benefits of a Graphical User Interface (GUI).
SaveIt​ is implemented for applying CS2103 learning outcomes and showing understanding of the software design. It is implemented by a group of CS2103 students, including myself. My key contribution is sort command and User Interface (UI) related with this function.
SaveIt is written in Java and JavaFX and is morphed from AddressBook-level4, the application used for teaching Software Engineering principles.
3. Summary of contributions
-
Major enhancement: Add the ability to sort issue list and UI of the sort type
-
What it does: It allows the user to sort issues shown in the list.
-
Justification: This feature improves the product significantly because it helps the user to view the issues in the order that they want so that they can organize the record of issues better. That can help users to search and learn more efficiently.
-
Highlights: This command changes the way how issues are listed in the application. It needs efforts to combine sorting and filtering together properly and make sure the correct issue can be retrieved from the sorted list. Backend structure also needs to be changed for comparing the issues in different order.
-
-
Minor enhancement:
-
Code contributed: [Functional code]
-
Other contributions:
4. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
4.1. Sorting the list of issues: (sr)sort
Sorts the list of issues according to a specified sort type.
The list always follows the specified sort type until another sort command is executed.
Format: sort
|
Format: sort chro
|
Format: sort freq
|
Format: sort tag
|
|
Examples:
4.2. Clearing all entries : (c)clear
Clears all issues and their solutions from SaveIt.
Format: clear
Example:
|
4.3. Choosing one primary solution: (sp)setprimary
Chooses one solution of the issue and make it the primary solution.
If there is an existing primary solution, setprimary
will reset the primary solution to the latest one.
The primary solution is shown on the top of the solution list and is highlighted in white.
Format: setprimary INDEX
|
4.4. Resetting the primary solution: (rp)resetprimary
Resets the primary solution and make all solutions normal and not highlighted.
Even if there is no primary solution, this command can still be executed, but no change will be shown.
Format: resetprimary
|
5. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
5.1. Sort feature
The sort command can sort an issue list shown in the GUI.
5.1.1. Current implementation
We use JavaFX SortedList
and the Comparator provided by SortType
to sort the list.
In order to allow sorting to work with the filtered list, we wrap FilteredList
with SortedList
and retrieve this new list with a new method getFilteredAndSortedList()
.
The following sequence diagram illustrates how the mechanism works:
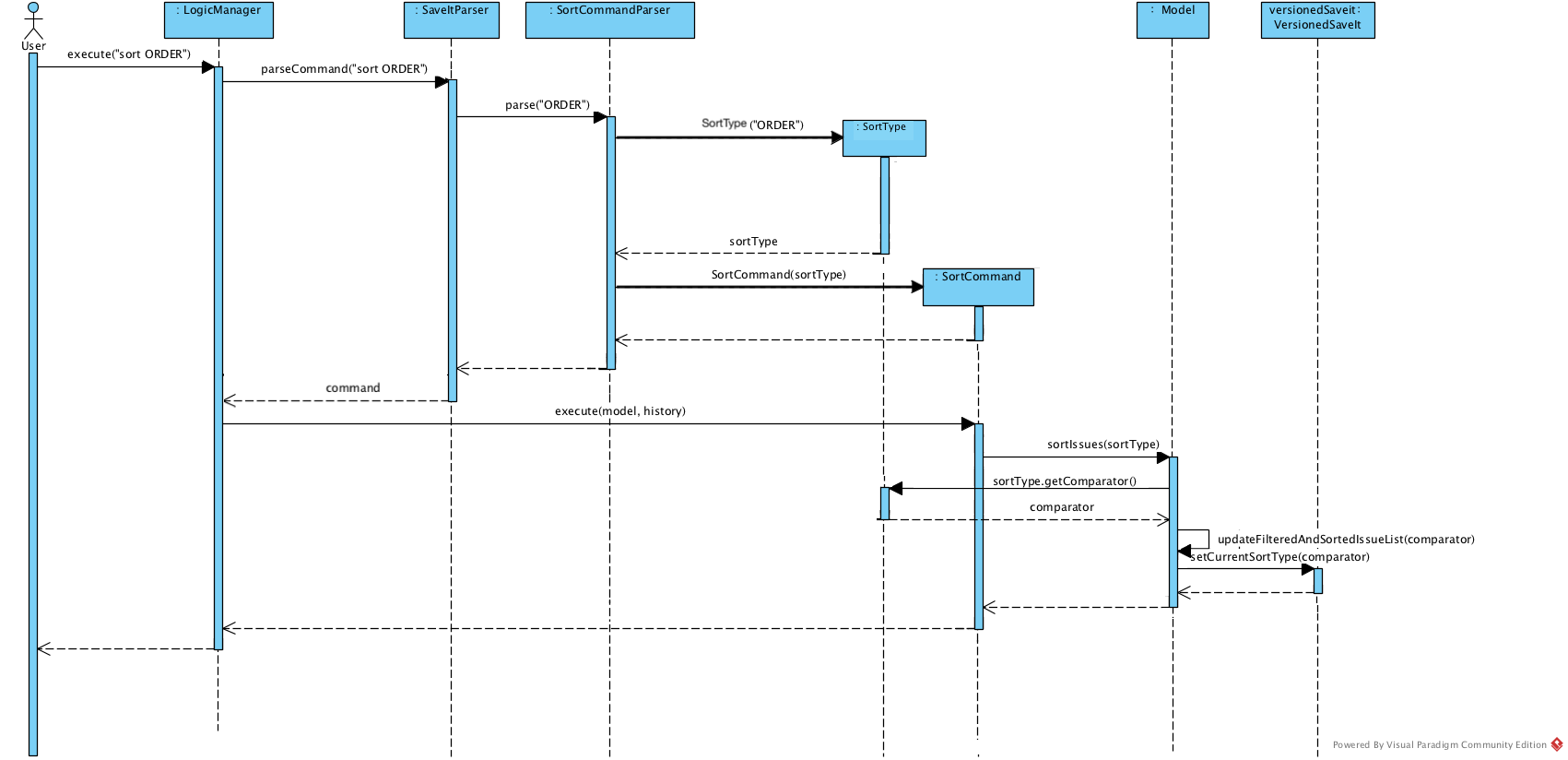
This diagram shows that after the input is parsed, a SortType object is initialized before the SortCommand object.
As shown in the diagram, a SortType object can provide the required comparator and we use the retrieved Comparator to sort the list when the sort command is executed.
The following is a class diagram for SortType.
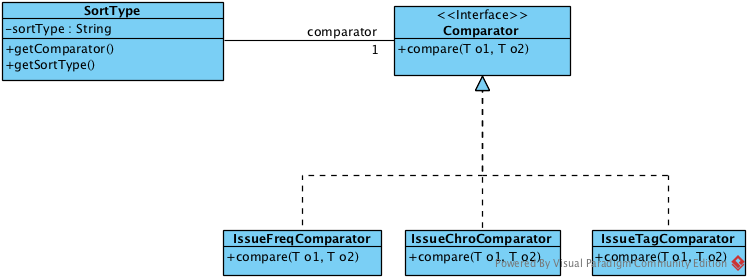
It shows that Comparator is an attribute of SortType class. We create three different classes that implement the Comparator Interface. They can serve the three sort types (excluding the default one) we provide in SaveIt. When more sort types are needed, we can simply create another class implementing Comparator, and add another case in the switch statement.
5.1.2. Design Considerations
Aspect: How sort executes
-
Alternative 1 (current choice): Combine SortedList and FilteredList, sort at GUI side
-
Pros: Consistent sorting. Doesn’t affect the memory of issue lists.
-
Cons: Need efforts to make sure the correct list is retrieved.
-
-
Alternative 2: Reconstruct UniqueIssueList directly, sort in Storage
-
Pros: Easy to understand and implement.
-
Cons: Break the persistence of the backend list.
-
5.2. Confirmation Check
Some commands that can affect the users' experience significantly need to be paid attention to and confirmation should be provided before they are executed.
For the current version, clear
is the only command that needs confirmation.
5.2.1. Current Implementation
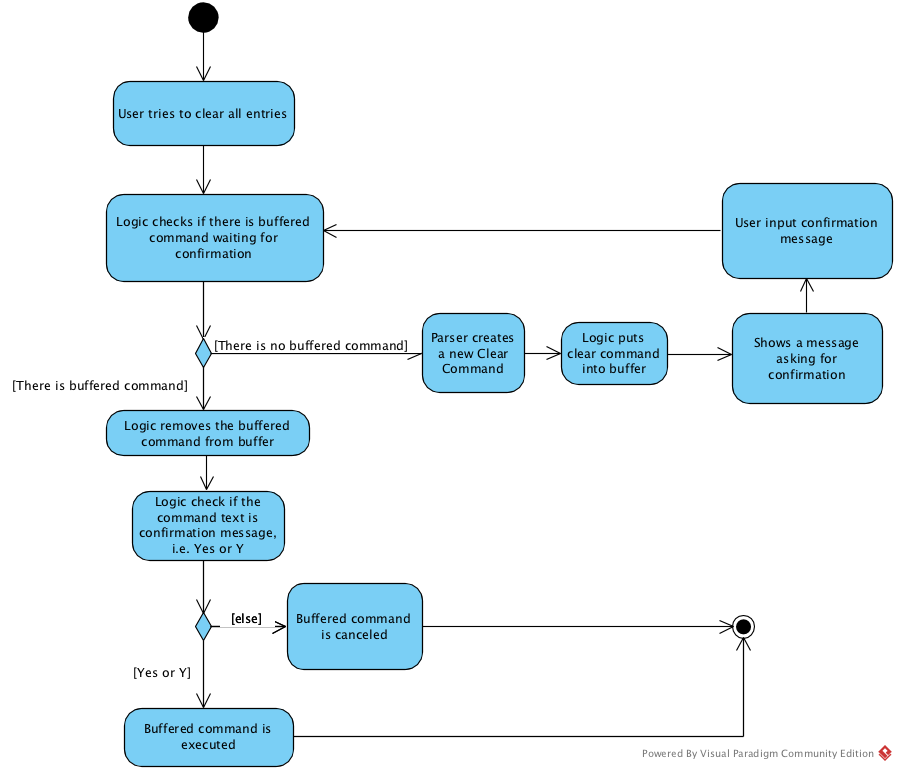
As shown in the diagram, instead of letting Logic execute the command directly after parsing it, we now check if we need confirmation before the command is executed.
In order to connect the confirmation with the command:
Step 1. We buffer the command that requires confirmation.
Step 2. Wait for the user to input confirmation message.
Step 3. Clear the buffer.
Step 4. Generate the CommandResult according to the confirmation message entered.
5.2.2. Design Consideration
Aspect: How to distinguish commands that need confirmation
-
Alternative 1 (current choice): Use abstract class DangerCommand
-
Pros: Protect the specific Command type from being accessed by LogicManager. Sustainable.
-
Cons: N.A.
-
-
Alternative 2: Check class name and provide a danger class name list
-
Pros: Easy to implement.
-
Cons: Command information leaked. Inaccurate, e.g. two classes from different packages can have the same class name.
-
Aspect: How to connect the confirmation message with the command requiring it
-
Alternative 1 (current choice): Buffer the command
-
Pros: Protect CommandBox from being accessed by Command, i.e. retrieve the input message in Command Class
-
Cons: Another variable introduced. Need efforts to deal with the buffered command properly.
-
-
Alternative 2: Let Command check the confirmation message
-
Pros: Follow the normal logic. Easy to understand.
-
Cons: CommandBox is exposed to Command Class.
-
5.3. Set Primary Feature
The set primary feature allows users to choose one solution as primary.
5.3.1. Current Implementation
The set primary solution mechanism is facilitated by the method isPrimarySolution()
, which is overrode by PrimarySolution
inheriting from Solution
.
5.3.2. Design Consideration
Aspect: How to distinguish between PrimarySolution and Solution
-
Alternative 1 (current choice): Use PrimarySolution as a subclass of Solution
-
Pros: No flag attribute is introduced. More sustainable.
-
Cons: New object is created. May affect performance.
-
-
Alternative 2: Add a flag attribute to Solution
-
Pros: Easy to understand and implement.
-
Cons: Solution constructor is affected. The flag attribute is redundant for non-primary solutions.
-
-
Alternative 3: Store the primary solution index in the solution list.
-
Pros: No new constructor and attribute are introduced.
-
Cons: Need to check if the index is affected every time when the solution list is updated.
-
5.4. Reset Primary Feature
The reset primary feature allows users to reset the primary solution and make all solutions normal and not highlighted.
5.4.1. Current Implementation
The reset primary solution mechanism goes through the solution list once and checks if a solution is primary or not. If it is primary, we replace it with a new Solution object with the same data of the primary solution. If it is not primary, we continue checking until we reach the end of the list.